当ソフトウェアは利用方法及びソースが読める方のみご利用いただけます。
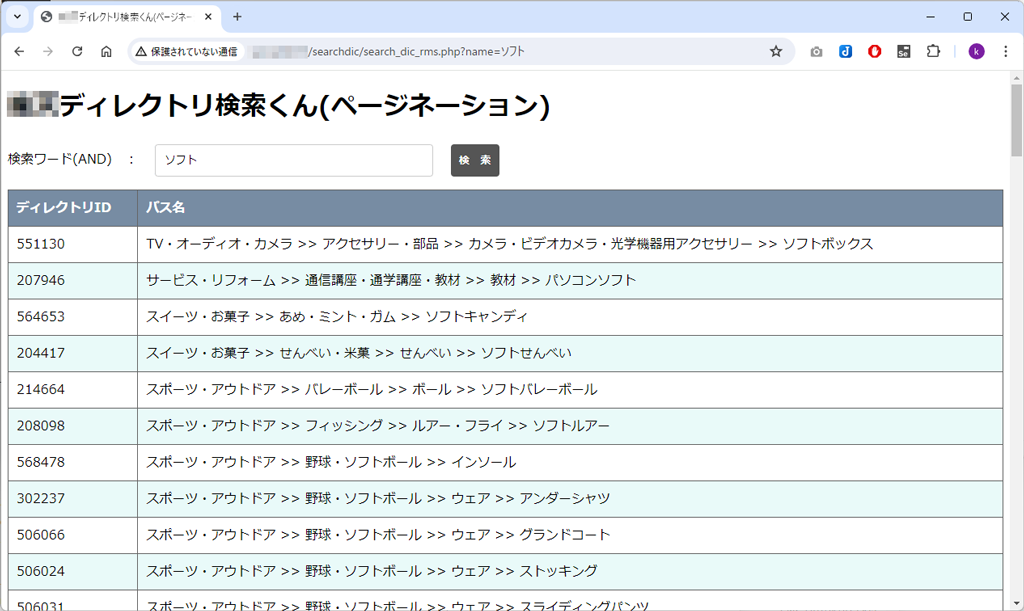
<?php
/**
* 汎用高速CSV検索くん
*
* CSVデータから検索ワードを含むものを表として高速に抽出します
* ページングしていないので対応レコード数はメモリに積めるだけです(MAX 1万件程度)
*
* 当ファイルを search.php として保存してください。PGは1ファイルのみです。
* 同フォルダに検索対象 search_db.csv を utf-8 ,改行LF ,tab区切り,タイトルあり
* で設置してください
*
* @version 1.0
* @category
* @package
* @author Takashi Kawahara <t_kawahara@chess-inc.com>
* @copyright (c) 2022 CHESS INC.
* @license (c) 2022 CHESS INC.Released under the GPLv3 license.
**/
// ------------------------------------------------------------------------
// 設定値
$CSV_DATA_FILE = "search_db.csv"; // CSVファイル1行目はタイトル必須です
$CSV_PARSER = "\t";
$TITLE = "汎用高速CSV検索くん";
// ------------------------------------------------------------------------
$scriptname = basename($_SERVER['SCRIPT_NAME']); // search.php
function h($str)
{
return htmlspecialchars($str, ENT_QUOTES, 'UTF-8');
}
$name = "";
if (isset($_GET['name'])) {
$name = h($_GET['name']);
}
?>
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8" />
<link rel="icon" href="data:;base64,=">
<title><?= $TITLE ?></title>
</head>
<body onload="load_focus()">
<h1><?= $TITLE ?></h1>
<form action="<?= $scriptname ?>" method="get">
<p>
検索ワード(含む) :
<input type="text" name="name" id="name" value="<?= $name; ?>" onchange="document.forms[0].submit();" class="form-text">
<input type="submit" value="検 索" class="form-submit-button">
</p>
</form>
<?php
$fp = fopen($CSV_DATA_FILE, 'r');
$total = 0;
$counter = 0;
$tableHtml = "<table>";
if ($line = fgetcsv($fp, 0, $CSV_PARSER)) {
// タイトル行
$tableHtml .= "<tr>";
foreach ($line as $col) {
$tableHtml .= "<th>{$col}</th>";
}
$tableHtml .= "</tr>";
while ($line = fgetcsv($fp, 0, $CSV_PARSER)) {
if (strpos(implode("\t", $line), $name) !== false) {
$tableHtml .= "<tr>";
foreach ($line as $col) {
$tableHtml .= "<td>{$col}</td>";
}
$tableHtml .= "</tr>";
$counter++;
}
$total++;
}
}
$tableHtml .= "</table>";
fclose($fp);
echo $tableHtml;
?>
<div style=" display: table; width: 100%;">
<p style="display: table-cell; text-align: left;">
</p>
<p style="display: table-cell; text-align: right;">
<?php echo "対象 " . number_format($counter) . " / 全 " . number_format($total) ?>
</p>
</div>
<style>
table {
width: 100%;
text-align: center;
border-collapse: collapse;
border-spacing: 0;
}
table tr:nth-child(2n+1) {
background: #e9faf9;
}
table th {
padding: 10px;
background: #778ca3;
border: solid 1px #666666;
color: #ffffff;
}
table td {
padding: 10px;
border: solid 1px #666666;
}
.form-text {
margin-right: 1rem;
width: 20rem;
height: 3em;
padding: 0 12px;
border-radius: 4px;
border: none;
box-shadow: 0 0 0 1px #ccc inset;
appearance: none;
-webkit-appearance: none;
-moz-appearance: none;
}
.form-text:focus {
outline: 0;
box-shadow: 0 0 0 2px rgb(33, 150, 243) inset;
}
.form-submit-button {
display: inline-block;
padding: 8px;
border: none;
border-radius: 4px;
background-color: #555;
color: #fff;
font-weight: bold;
appearance: none;
-webkit-appearance: none;
-moz-appearance: none;
cursor: pointer;
border: 2px solid transparent;
}
.form-submit-button:hover {
background-color: #aaa;
}
.form-submit-button:focus {
outline: 0;
background-color: #555;
border: 2px solid rgb(33, 150, 243);
}
</style>
<script>
// 初期オープン時のINPUTのTEXT位置を設定
function load_focus() {
var elm = document.getElementById('name');
var val = elm.value;
elm.value = '';
elm.focus();
elm.value = val;
}
</script>
</body>
</html>